WDI (WLAN Device Driver Interface) is the new Universal Windows driver model for Windows 10. WLAN device manufacturers can write a single WDI miniport driver that runs on all device platforms, and requires less code than the previous native WLAN driver model. All new WLAN features introduced in Windows 10 require WDI-based drivers.

Programming a device driver for Linux requires a deep understanding of the operating system and strong development skills. To help you master this complex domain, Apriorit driver development experts created this tutorial.
- Fix Modem Drivers problems. Modem driver problems, although pretty common, are easy to resolve. Read on to learn about the more common modem drivers problems, and how you can fix these problems quickly and safely.
- Install the driver to establish a cable connection between your compatible Nokia phone and a compatible PC.Important for Windows 2000 or XP or Vista users: Drivers for the Nokia DKE-2, DKU-2, CA-42, CA-53, CA-70, and CA-101 cables are now.
We’ll show you how to write a device driver for Linux (5.3.0 version of the kernel). In doing so, we’ll discuss the kernel logging system, principles of working with kernel modules, character devices, the file_operations structure, and accessing user-level memory from the kernel. You’ll also get code for a simple Linux driver that you can augment with any functionality you need.
This article will be useful for developers studying Linux driver development.
Contents:
Getting started with the Linux kernel module
The Linux kernel is written in the C and Assembler programming languages. C implements the main part of the kernel, while Assembler implements architecture-dependent parts. That’s why we can use only these two languages for Linux device driver development. We cannot use C++, which is used for the Microsoft Windows kernel, because some parts of the Linux kernel source code (e.g. header files) may include keywords from C++ (for example, delete
or new
), while in Assembler we may encounter lexemes such as ‘ : : ’
.
There are two ways of programming a Linux device driver:
- Compile the driver along with the kernel, which is monolithic in Linux.
- Implement the driver as a kernel module, in which case you won’t need to recompile the kernel.
In this tutorial, we’ll develop a driver in the form of a kernel module. A module is a specifically designed object file. When working with modules, Linux links them to the kernel by loading them to the kernel address space.
Module code has to operate in the kernel context. This requires a developer to be very attentive. If a developer makes a mistake when implementing a user-level application, it will not cause problems outside the user application in most cases. But mistakes in the implementation of a kernel module will lead to system-level issues.

Luckily for us, the Linux kernel is resistant to non-critical errors in module code. When the kernel encounters such errors (for example, null pointer dereferencing), it displays the oops
message — an indicator of insignificant malfunctions during Linux operation. After that, the malfunctioning module is unloaded, allowing the kernel and other modules to work as usual. In addition, you can analyze logs that precisely describe non-critical errors. Keep in mind that continuing driver execution after an oops
message may lead to instability and kernel panic.
The kernel and its modules represent a single program module and use a single global namespace. In order to minimize the namespace, you must control what’s exported by the module. Exported global characters must have unique names and be cut to the bare minimum. A commonly used workaround is to simply use the name of the module that’s exporting the characters as the prefix for a global character name.
With this basic information in mind, let’s start writing our driver for Linux.
Creating a kernel module
Update Modem Drivers
We’ll start by creating a simple prototype of a kernel module that can be loaded and unloaded. We can do that with the following code:
Most people connect to internet through cable modems, DSL, or fiber optics. No matter what type of modem you use, they all do modulation and demodulation. This is why modem is also called “modulator-demodulator”. Modem acts as a translator so that signals from your computer can travel over phone lines. It’s analogous to two people wanting to talk and having uncommon language. They will definitely need a translator. Translator knows both the languages and translates each one of them for both the parties so that both can understand and communicate. Rather than literally translating, modem translates signals from your computer to phone lines, DSL, or fiber optics so to bridge the communication between the two. In order to be completely functional, you need to update modem drivers if need be.


Modem Drivers are set of codes that receive signals from your computer, make packets of that data for modem and transmit it over phone lines, DSL, or fiber optics even in the air. Moreover, it decodes data packets received from any location of the world so that it could be understandable for your computer. These are modem drivers through which you enjoy the facility of email or internet. You can use driver updates to keep your modem driver up-to-date which are available on one-click access free of cost.
Sometimes, you may face problems due to modem drivers. The reasons can be:
• You installed a new modem
• Recently upgraded your operating systems
• Modem driver has been corrupted by undesired application or system Crash etc.
Windows 10 Modem Driver
How to update modem drivers?
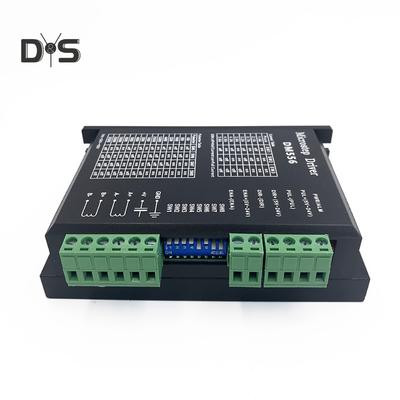
Windows 7 Modem Drivers Download
Facing a problem in modem drivers is not a serious problem. One can easily fix it by re-installing or updating the modem driver. There are typically three ways to update modem driver:
• Install Windows updates which might install the latest drivers for your modem
• Open Device manager from control panel and update driver modem
• Open manufacturer’s website and find compatible modem driver for your modem
For detailed steps and illustration of how to update modem driver, check out “How to install/ update drivers?”
